면접에서 batch normalization이 training하기 전에 실행하냐? training하면서 실행하냐? 이런걸 물어봤는데
batch normalization에 대해 몇가지를 배워보자
1. 핵심 아이디어
The idea is that, instead of just normalizing the inputs to the network, we normalize the inputs to layers within the network.
network에 대한 input을 normalizing하는 것 대신에 network 내부의 layer에 대한 input을 normalizing함
It's called batch normalization because during training, we normalize each layer's inputs by using the mean and variance of the values in the current batch.
batch normalization이라고 불리는 이유는... training하는 동안에 현재 batch의 mean,variance를 이용하여 각 layer로 들어가는 input을 normalizing하기 때문
그러니까 training 하기 전에 batch normalization을 미리 다 해놓는게 아니라
training중에 사용하는 batch들의 mean, variance로 normalizing해서 다음 layer들의 input으로 넣어주는거지
https://deepdata.tistory.com/886
neural network를 training 하기 전에 input을 normalization해야하는 이유
1. normalization 신경망의 훈련을 빠르게 하기 위해 필요한 input normalization 다음과 같이 2차원의 입력데이터가 존재할때 주어진 데이터의 평균을 빼고, 표준편차를 나누는 방법으로 normalization할 수
deepdata.tistory.com
input을 normalization하는 이유는 cost function을 optimization하기 쉽기 때문이다.
2. 중요 디테일
1) batch normalization을 사용하는 layer는 bias를 포함시키지 않는다
2) linear layer의 output에 대한 batch normalization은 nn.BatchNorm1d
convolutional layer의 output인 filtered image같은 2d output은 nn.BatchNorm2d
3) activation function을 사용하기 전에 batch normalization layer를 통과시킨다.
linear layer > batch normalization layer > activation > linear > ...
import torch.nn as nn
import torch.nn.functional as F
class NeuralNet(nn.Module):
def __init__(self, use_batch_norm, input_size = 784, hidden_dim = 256, output_size = 10):
"""
Creates a PyTorch net using the given parameters.
:param use_batch_norm: bool
Pass True to create a network that uses batch normalization; False otherwise
Note: this network will not use batch normalization on layers that do not have an
activation function.
"""
super(NeuralNet, self).__init__() #init super
#default layer sizes
self.input_size = input_size #(28*28 images)
self.hidden_dim = hidden_dim
self.output_size = output_size #(number of classes)
#keep track of whether or not this network uses batch normalization
self.use_batch_norm = use_batch_norm
# define hidden linear layers, with optional batch norm on their outputs
# layers with batch_norm applied have no bias term
if use_batch_norm:
self.fc1 = nn.Linear(input_size, hidden_dim*2, bias = False)
self.batch_norm1 = nn.BatchNorm1d(hidden_dim*2)
else:
self.fc1 = nn.Linear(input_size, hidden_dim*2)
# define *second* hidden linear layers, with optional batch norm on their outputs
if use_batch_norm:
self.fc2 = nn.Linear(hidden_dim*2, hidden_dim, bias = False)
self.batch_norm2 = nn.BatchNorm1d(hidden_dim)
else:
self.fc2 = nn.Linear(hidden_dim*2, hidden_dim)
## third and final, fully-connected layer
self.fc3 = nn.Linear(hidden_dim, output_size)
def forward(self, x):
#flatten image
x = x.view(-1, 28*28)
# all hidden layers + optional batch norm + relu activation
x = self.fc1(x)
if self.use_batch_norm:
x = self.batch_norm1(x)
x = F.relu(x)
#second layer
x = self.fc2(x)
if self.use_batch_norm:
x = self.batch_norm2(x)
x = F.relu(x)
#third layer, no batch norm or activation
x = self.fc3(x)
return x
3. 성능 비교
동일한 multi layer perceptron을 MNIST dataset으로 training할 때 batch normalization layer를 사용하느냐?
사용하지 않느냐에 따라 training loss 자체가 다르다
batch normalization을 사용했을때 training이 효과적이다.
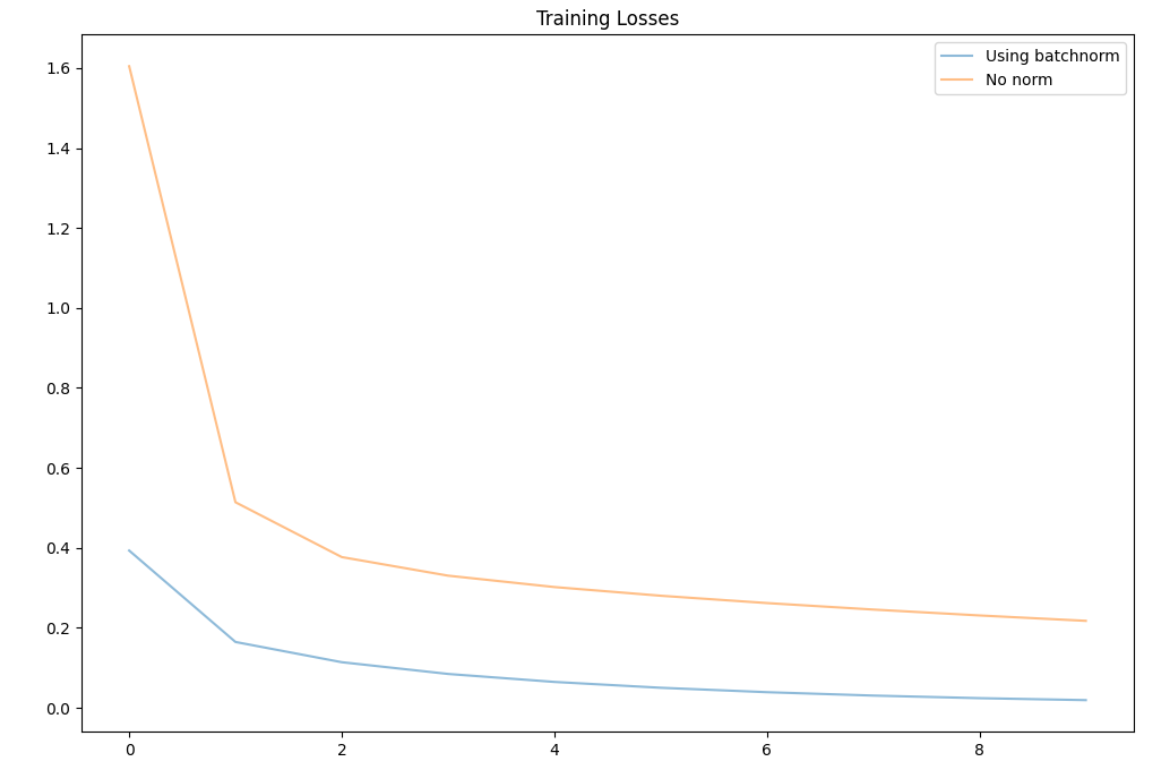
4. model.train(), model.eval()
batch normalization은 매우 큰 데이터셋일수록 training과 test/evaluation에서 다른 행동을 보이기 때문에
반드시 model.train(), model.eval()을 사용해야함
test과정에서 model.train()상태에서 test를 하고 model.eval()에서 test를 해보면...
model.eval()에서 약간 더 성능이 좋았다.
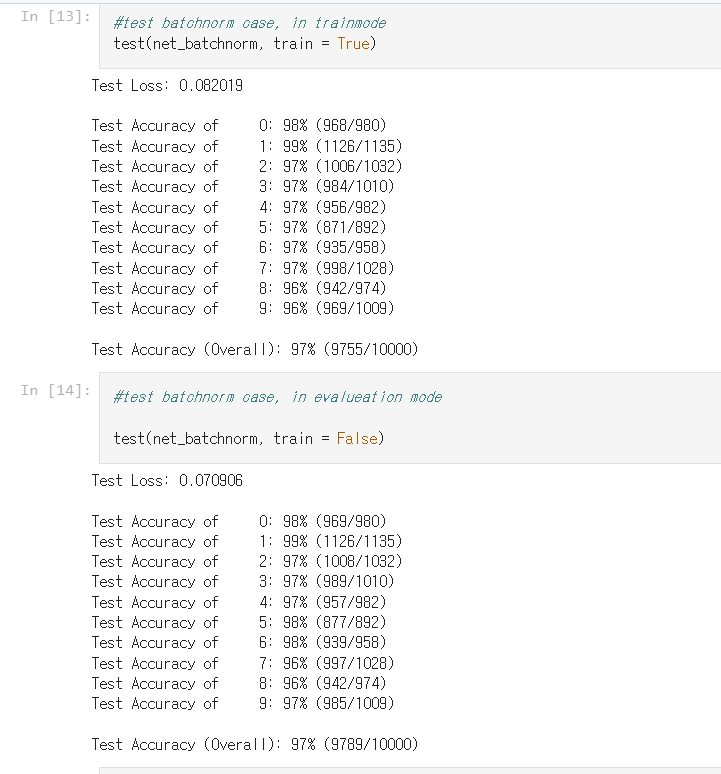
Training mode means that the batch normalization layers will use batch statistics to calculate the batch norm.
Evaluation mode, on the other hand, uses the estimated population mean and variance from the entire training set, which should give us increased performance on this test data.
training mode에서 batch normalization은 batch norm을 계산하기 위해 batch 통계량을 사용하고
evaluation mode에서 batch normalization은 전체 training set으로부터 추정된 모집단의 평균 분산을 사용하여 test data에서 향상된 성능을 보여준다.
batch normalization을 쓰지 않는 경우 test 성능도 쓰는 경우에 비해 떨어진다.
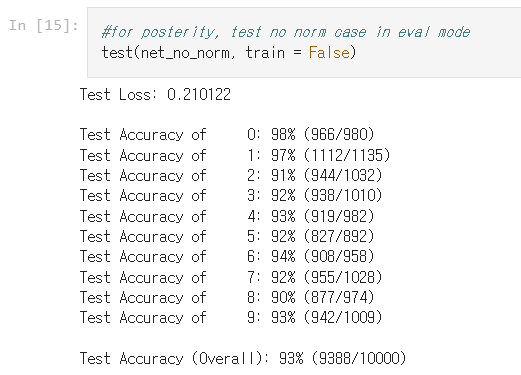
'딥러닝 > 딥러닝 기초' 카테고리의 다른 글
reproducibility를 위한 random seed (0) | 2023.12.11 |
---|---|
구현하면서 배우는 weight initialization(가중치 초기화) 중요성 (0) | 2023.09.13 |
컴퓨터로 sound data를 표현하는 방법들에 대하여(Fourier transform, spectrogram, melspectrogram, MFCC) (0) | 2023.07.05 |
Nearest neighbor search vs. t-sne를 이용한 차원 축소 기법 (0) | 2023.06.18 |
자연어 처리 기술의 또 다른 혁명 - ChatGPT 시대 우리는 무엇을 해야하는가 - (0) | 2023.06.02 |