1. 2차원 배열 선언
정수형 2차원 배열은 new int[행 개수][열 개수];로 선언 가능하다
int[][] arr2d = new int[4][4];
2차원 격자상 좌표가 (x,y)라고 한다면.. y행 x열에 대한 원소 접근은 arr2d[y][x];와 같이 접근할 수 있다.
/*
i/j 0 1 2 3
0 1 2 3 4
1 7 8 9 10
2 11 12 13 14
3 15 16 17 18
*/
public class Main {
public static void main(String[] args) {
int[][] arr2d = new int[][]{
{1, 2, 3, 4},
{7, 8, 9, 10},
{11, 12, 13, 14},
{15, 16, 17, 18}
};
System.out.println(arr2d[0][0]); // (0,0)
System.out.println(arr2d[1][2]); // (2,1)
}
}
원소 바꾸는 것도 당연히 가능하다.
(0,0)원소를 15로 바꾸고 싶다면 arr2d[0][0] = 15;로 바꾸면 된다
public class Main {
public static void main(String[] args) {
int[][] arr2d = new int[][]{
{1, 2, 3, 4},
{7, 8, 9, 10},
{11, 12, 13, 14},
{15, 16, 17, 18}
};
System.out.println(arr2d[0][0]); // 1행 1열 -> 1
arr2d[0][0] = 15;
System.out.println(arr2d[0][0]); // 1행 1열 -> 15
}
}
주어진 2차원 배열을 0열부터 행방향으로 차례대로 순회하는 코드는..
파이썬에서 하던대로 하면 된다
import java.util.Scanner;
public class Main {
public static void main(String[] args) {
// 여기에 코드를 작성해주세요.
Scanner sc = new Scanner(System.in);
int[][] maps = new int[4][4];
for (int y=0; y < 4; y++){
for(int x = 0; x < 4; x++){
maps[y][x] = sc.nextInt();
}
}
for(int y = 0; y < 4; y++){
for(int x = 0; x < 4; x++){
System.out.print(maps[y][x] + " ");
}
System.out.println();
}
}
}
입력
1 2 3 4
7 8 9 10
11 12 13 14
15 16 17 18
출력
1 2 3 4
7 8 9 10
11 12 13 14
15 16 17 18
2. 2차원 배열 오른쪽으로 90도 회전
외우는건 아니고 각 위치가 어떻게 오는지 확인해서 인덱스를 적절하게 설정하기
(0,2)인 7이 (0,0)
(0,1)인 4가 (1,0)
(0,0)인 1이 (2,0)으로 오니까...
arr[2-x][y]로 하면 될 것 같다
int[][] arr = new int[][]{ {1, 2, 3}, {4, 5, 6}, {7, 8, 9} };
for (y = 0; y < 3; i++) {
for(x = 0; x < 3; j++) {
System.out.print(arr[2-x][y] + " ");
}
System.out.println();
}
입력
1 2 3
4 5 6
7 8 9
출력결과
7 4 1
8 5 2
9 6 3
3. 대각선 아래 원소들의 합
대각선 부분은 y=x일때이고, 대각선 아래는 y > x이고 대각선 위는 y < x

import java.util.Scanner;
public class Main {
public static void main(String[] args) {
// 여기에 코드를 작성해주세요.
Scanner sc = new Scanner(System.in);
int[][] arr = new int[4][4];
for(int y = 0; y < 4; y++){
for(int x = 0; x < 4; x++){
arr[y][x] = sc.nextInt();
}
}
int sum = 0;
for(int y = 0; y < 4; y++){
for(int x = 0; x < 4; x++){
if(y >= x){
sum += arr[y][x];
}
}
}
System.out.println(sum);
}
}
4. 2차원 배열 초기화
자바는 정수형 2차원 배열을 정의하기만 해도 전부 숫자 0으로 채워넣은 배열이 생성된다
public class Main {
public static void main(String[] args) {
int[][] arr2d = new int[4][4];
int n = 4;
for (int i = 0; i < n; i++) {
for (int j = 0; j < n; j++) {
System.out.print(arr2d[i][j] + " ");
}
System.out.println();
}
}
}
>> 출력 결과
0 0 0 0
0 0 0 0
0 0 0 0
0 0 0 0
5. 지그재그로 채우기
당연히 외우는게 아니고 보면서 구현한다는 생각으로

짝수번째 x열에서는 y가 증가하는 방향이고 홀수번째 x열에서는 y가 감소하는 방향이니까..
import java.util.Scanner;
public class Main {
public static void main(String[] args) {
// 여기에 코드를 작성해주세요.
Scanner sc = new Scanner(System.in);
int n = sc.nextInt();
int m = sc.nextInt();
int[][] arr = new int[n][m];
int num = 0;
for(int x = 0; x < m; x++){
if(x % 2 == 0){
for(int y = 0; y < n; y++){
arr[y][x] = num;
num++;
}
} else {
for(int y = n-1; y >= 0; y--){
arr[y][x] = num;
num++;
}
}
}
for(int y = 0; y < n; y++){
for(int x = 0; x < m; x++){
System.out.print(arr[y][x] + " ");
}
System.out.println();
}
}
}
4 2
0 7
1 6
2 5
3 4
그러면 (n-1,n-1)부터 시작해서 반대방향으로 지그재그로 채우고 싶으면 어떻게 해야할까?
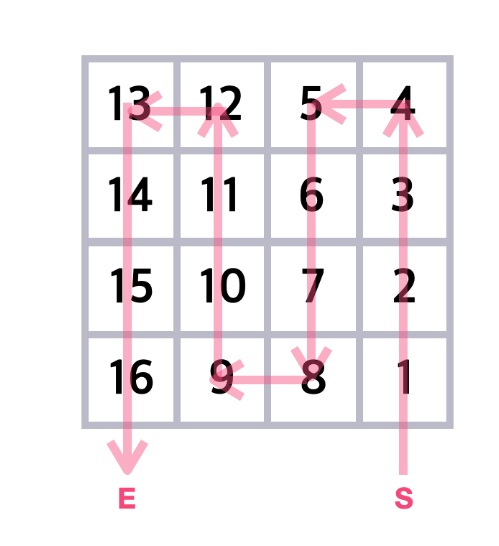
i라는 지시변수를 생성해서 1부터 시작해서 i가 홀수이면 y가 감소하는 방향이고
i가 짝수이면 y가 증가하는 방향
x열은 n-1부터 시작해서 0까지 감소하는 방향이고...
import java.util.Scanner;
public class Main {
public static void main(String[] args) {
// 여기에 코드를 작성해주세요.
Scanner sc = new Scanner(System.in);
int n = sc.nextInt();
int[][] arr = new int[n][n];
int num = 1;
int i = 1;
for(int x = n-1; x >= 0; x--){
if(i % 2 == 1){
for(int y = n-1; y >= 0; y--){
arr[y][x] = num;
num++;
}
} else {
for(int y = 0; y < n; y++){
arr[y][x] = num;
num++;
}
}
i++;
}
for(int y = 0; y < n; y++){
for(int x = 0; x < n; x++){
System.out.print(arr[y][x] + " ");
}
System.out.println();
}
}
}
4
13 12 5 4
14 11 6 3
15 10 7 2
16 9 8 1
6. 오른쪽 아래 대각선으로 숫자 채우기
이건 중요한 테크닉이라 외우긴 해야할듯
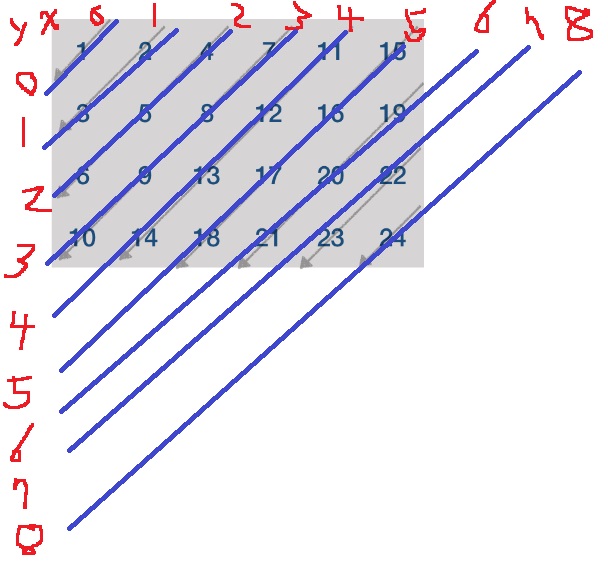
배열에서 좌표가 (x,y)이면.. 오른쪽 아래 사선방향으로는 x+y가 일정하다
i (=x+y)를 0부터 n+m까지 증가시키고
y,x로 순회하면서 x+y가 i와 같다면 숫자를 채워넣는 방식으로
import java.util.Scanner;
public class Main {
public static void main(String[] args) {
// 여기에 코드를 작성해주세요.
Scanner sc = new Scanner(System.in);
int n = sc.nextInt();
int m = sc.nextInt();
int[][] arr = new int[n][m];
int num = 1;
for(int i = 0; i <= n+m; i++){
for(int y = 0; y < n; y++){
for(int x = 0; x < m; x++){
if(x+y == i){
arr[y][x] = num;
num++;
}
}
}
}
for(int y = 0; y < n; y++){
for(int x = 0; x < m; x++){
System.out.print(arr[y][x] + " ");
}
System.out.println();
}
}
}
3 3
1 2 4
3 5 7
6 8 9
참고로 왼쪽 위에서 오른쪽 아래로 내려가는 반대방향 사선은 x-y가 일정할거
7. 파스칼의 삼각형 만들기
파이썬과 다르게 뭔가 힘든것 같냐..
n*n 배열을 생성하고 (0,0)을 1로 채우고
각 행의 처음과 끝을 1로 채운다.
y = 2행부터 시작해서, 각 행은 1열부터 y-1열까지 채우기 시작한다.
(x,y)의 원소는 바로 위 행인 y-1행의 x-1열, x열 원소의 합이다.
arr[y][x] = arr[y-1][x] + arr[y-1][x-1]
import java.util.Scanner;
public class Main {
public static void main(String[] args) {
// 여기에 코드를 작성해주세요.
Scanner sc = new Scanner(System.in);
int n = sc.nextInt();
int[][] arr = new int[n][n];
arr[0][0] = 1;
for(int y = 1; y < n; y++){
arr[y][0] = 1;
arr[y][y] = 1;
}
for(int y = 2; y < n; y++){
for(int x = 1; x < y; x++){
arr[y][x] = arr[y-1][x-1] + arr[y-1][x];
}
}
for(int y = 0; y < n; y++){
for(int x = 0; x <n; x++){
if(y >= x){
System.out.print(arr[y][x] + " ");
}
}
System.out.println();
}
}
}
6
1
1 1
1 2 1
1 3 3 1
1 4 6 4 1
1 5 10 10 5 1
8. visited 배열
2차원 배열 만들어서 해당 점의 위치가 존재한다면 1을 표시하면 된다
import java.util.Scanner;
public class Main {
public static void main(String[] args) {
// 여기에 코드를 작성해주세요.
Scanner sc = new Scanner(System.in);
int n = sc.nextInt();
int m = sc.nextInt();
int[][] arr = new int[n][n];
for(int i = 0; i < m; i++){
int r = sc.nextInt();
int c = sc.nextInt();
arr[r-1][c-1] = 1;
}
for(int y = 0; y < n; y++){
for(int x = 0; x < n; x++){
System.out.print(arr[y][x] + " ");
}
System.out.println();
}
}
}
2 3
1 1
1 2
2 2
1 1
0 1
'알고리즘 > Java 기초' 카테고리의 다른 글
자바 자료구조3 -자바에서 스택, 큐, 덱 사용법- (0) | 2023.03.04 |
---|---|
자바 초보부터 B형까지9 -class 생성하기 필수- (0) | 2023.03.01 |
자바 초보부터 B형까지7 -변수의 범위, 지역변수, 전역변수 설정방법- (0) | 2023.03.01 |
자바 초보부터 B형까지6 -함수 작성법 필수- (0) | 2023.03.01 |
자바 초보에서 B형까지5 -문자열 필수지식- (0) | 2023.02.26 |