1. foreach
배열이나 컬렉션에서 모든 원소 각각을 한번씩 꺼내서 쭉 써보겠다는 의미의 반복문
foreach ((데이터타입) (변수명) in (컬렉션명)){반복 실행할 명령}
1) 배열
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class HelloWorld : MonoBehaviour
{
// Start is called before the first frame update
void Start()
{
int[] a = { 2, 4, 6, 8, 10 };
foreach (int number in a)
{
Debug.Log(number);
}
}
// Update is called once per frame
void Update()
{
}
}
파이썬에서 리스트에서 원소 직접 꺼내서
for a in A: 이렇게 하듯이, 원소를 index로 지정하지 않고 직접 꺼내는 것과 같다
number 자체가 a 배열 내의 원소들 반복 1회마다 하나씩을 가리킨다
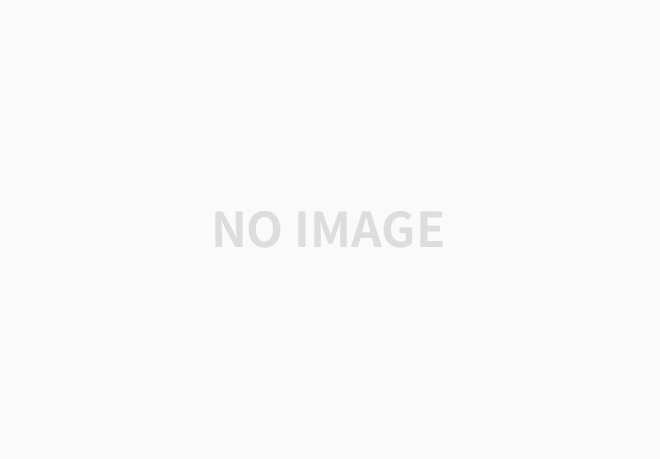
2) 다차원 배열
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class HelloWorld : MonoBehaviour
{
// Start is called before the first frame update
void Start()
{
int[,] a = { { 2, 4, 6, 8, 10 },
{1,2,3,4,5 },
{2,3,4,5,6 },
{6,7,8,9,10 }
};
foreach (int number in a)
{
Debug.Log(number);
}
}
// Update is called once per frame
void Update()
{
}
}
2,4,6,8,10
1,2,3,4,5
2,3,4,5,6
6,7,8,9,10
이렇게 되어 있으면.. 2 > 4 > 6 > 8 > 10 > 1 > 2 > 3 > 4 > 5 ....이렇게 하나씩 돈다
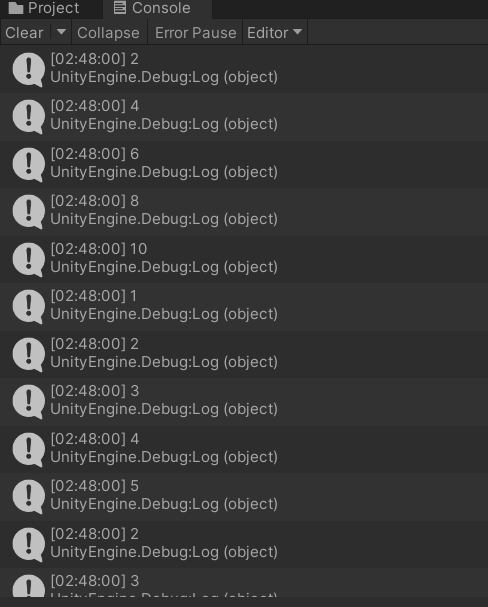
3) 가변 배열
a에서 하나씩 꺼내오는데 int number로 취해서 에러가 난다
a 배열의 원소 하나하나가 크기 5인 배열인데 int로 해서

int number말고 int[] number로 하면 에러가 안난다
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class HelloWorld : MonoBehaviour
{
// Start is called before the first frame update
void Start()
{
int[][] a = new int[5][];
a[0] = new int[5] { 1, 2, 3, 4, 5 };
a[1] = new int[5] { 6, 7, 8, 9, 10 };
a[2] = new int[5] { 7, 2, 3, 1, 5 };
a[3] = new int[5] { 9, 1, 2, 1, 5 };
a[4] = new int[5] { 4, 1, 2, 6, 3 };
foreach (int[] number in a)
{
Debug.Log(number);
}
}
// Update is called once per frame
void Update()
{
}
}

각 배열내의 원소를 보고 싶으면...
foreach (int[] number in a) {
for (int i = 0; i < number.Length; i++){
Debug.Log(number[i]);
}
}
이런식으로 각 배열을 또 순회해줘야함
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class HelloWorld : MonoBehaviour
{
// Start is called before the first frame update
void Start()
{
int[][] a = new int[5][];
a[0] = new int[5] { 1, 2, 3, 4, 5 };
a[1] = new int[5] { 6, 7, 8, 9, 10 };
a[2] = new int[5] { 7, 2, 3, 1, 5 };
a[3] = new int[5] { 9, 1, 2, 1, 5 };
a[4] = new int[5] { 4, 1, 2, 6, 3 };
foreach (int[] number in a)
{
foreach (int n in number)
{
Debug.Log(n);
}
}
}
// Update is called once per frame
void Update()
{
}
}

4) List
1차원 배열이랑 다를 것이 없는
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class HelloWorld : MonoBehaviour
{
// Start is called before the first frame update
void Start()
{
List<string> names = new List<string>();
names.Add("젤다");
names.Add("링크");
names.Add("페르소나");
foreach (string name in names)
{
Debug.Log(name);
}
}
// Update is called once per frame
void Update()
{
}
}
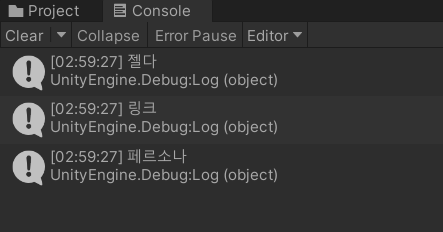
5) Dictionary
Dictionary는 key - value를 저장하는 자료구조이기 때문에, foreach로 가져오는 방법이 2가지 있다
1가지는 KeyValuePair<string,int> pair라는 KeyValuePair라는 데이터 타입으로
key,value를 쌍으로 가져옴
pair에는 속성으로 pair.Key, pair.Value가 있고 각각 key, value를 가져온다
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class HelloWorld : MonoBehaviour
{
// Start is called before the first frame update
void Start()
{
Dictionary<string,int> points = new Dictionary<string,int>();
points.Add("슬라임", 100);
points.Add("츄츄족", 200);
points.Add("우인단", 300);
points.Add("고래", 1000);
foreach(KeyValuePair<string,int> pair in points)
{
Debug.Log(pair.Key + ": " + pair.Value);
}
}
// Update is called once per frame
void Update()
{
}
}

다른 방법으로, points.Keys하면 points라는 딕셔너리에서 key들만 모은 컬렉션을 구하는데,
여기서 foreach를 하면 points에 존재하는 key만 가져올 수 있다
key를 알면, value를 points[key]로 key에 대응하는 value를 구할 수 있다
참고로 당연히 Keys가 있으면 value들을 모아놓은 Values도 있긴 있다
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class HelloWorld : MonoBehaviour
{
// Start is called before the first frame update
void Start()
{
Dictionary<string,int> points = new Dictionary<string,int>();
points.Add("슬라임", 100);
points.Add("츄츄족", 200);
points.Add("우인단", 300);
points.Add("고래", 1000);
foreach (string key in points.Keys)
{
Debug.Log(key + ": " + points[key]);
}
}
// Update is called once per frame
void Update()
{
}
}
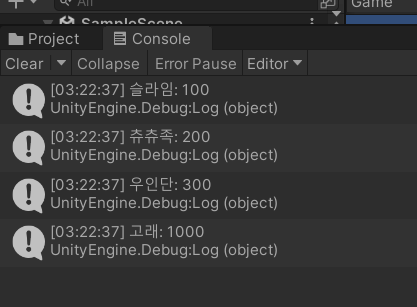
2. 연습문제1
초기값 a, 공비 b에 대해 등비수열 10항 출력
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class HelloWorld : MonoBehaviour
{
// Start is called before the first frame update
void Start()
{
int a = 3;
int b = 2;
for (int i = 1; i <= 10; i++)
{
Debug.Log(a);
a *= b;
}
}
// Update is called once per frame
void Update()
{
}
}
3. 연습문제2
2차원 가변배열을 만들어 정수를 저장
이 가변배열에서 10이 넘는 수만을 새로 만든 리스트에 저장
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class HelloWorld : MonoBehaviour
{
// Start is called before the first frame update
void Start()
{
int[][] a = new int[3][];
a[0] = new int[5] { 3,11,12,1,5};
a[1] = new int[4] { 13, 11, 2, 5 };
a[2] = new int[3] { 9, 7, 1 };
List<int> list = new List<int>();
foreach (int[] number in a)
{
foreach (int n in number)
{
if(n > 10)
{
list.Add(n);
}
}
}
foreach (int n in list)
{
Debug.Log(n);
}
}
// Update is called once per frame
void Update()
{
}
}
4. 연습문제3
크기 10의 정수배열에서 원하는 수를 넣고, 이 배열을 뒤집는다.
배열의 길이 파이썬의 len()함수에 대응하는 것은 a.Length가 있다.
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class HelloWorld : MonoBehaviour
{
// Start is called before the first frame update
void Start()
{
int[] a = new int[10];
for (int i = 0; i < a.Length; i++)
{
a[i] = 2 * i + 1;
}
for (int i = 0; i < a.Length/2; i++)
{
int b = a[i];
a[i] = a[a.Length - i - 1];
a[a.Length - i - 1] = b;
}
foreach (int n in a)
{
Debug.Log(n);
}
}
// Update is called once per frame
void Update()
{
}
}
5. 연습문제 4
5*5 bool 배열에서 (i,j) 원소를 i > j이면 true, i <= j이면 false로 채운다
참고로 처음에 bool 배열의 기본값이 false라서, false는 채우지 않아도 된다
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class HelloWorld : MonoBehaviour
{
// Start is called before the first frame update
void Start()
{
bool[,] a = new bool[5, 5];
for (int i = 0; i < 5; i++)
{
for (int j = 0; j < 5; j++)
{
if(i > j)
{
a[i, j] = true;
}
else
{
a[i, j] = false;
}
}
}
}
// Update is called once per frame
void Update()
{
}
}
6. 연습문제5
1부터 1000까지의 수 중에서 0과 5로만 이루어진 수를 찾아 List에 저장하고 출력
.ToString()으로 하면 string으로 바꿀 수 있는데
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class HelloWorld : MonoBehaviour
{
// Start is called before the first frame update
void Start()
{
List<string> list = new List<string>();
for (int i = 1; i <= 1000; i++)
{
string s = i.ToString();
if (s.Contains("1") || s.Contains("2") || s.Contains("3") || s.Contains("4") || s.Contains("6") || s.Contains("7") || s.Contains("8") || s.Contains("9"))
{
continue;
}
else
{
list.Add(s);
}
}
foreach(string s in list)
{
Debug.Log(s);
}
}
// Update is called once per frame
void Update()
{
}
}
ToString()없이 할려면 현재 수 i의 일의자리, 10의자리, 100의자리,...가 0이나 5인지 체크하면 된다
반복적으로 10으로 나눠서 확인하면 되는데.. 생각을 못했네
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class HelloWorld : MonoBehaviour
{
// Start is called before the first frame update
void Start()
{
List<int> list = new List<int>();
for (int i = 1; i < 1000; i++)
{
bool flag = true;
int x = i;
while (x > 0) {
int y = x % 10;
if (y != 0 && y != 5)
{
flag = false;
break;
}
x /= 10;
}
if (flag == true)
{
list.Add(i);
}
}
foreach (int n in list)
{
Debug.Log(n);
}
}
// Update is called once per frame
void Update()
{
}
}
7. 연습문제 6
몬스터이름 : 경험치로 만들어지는 dictionary를 만들고, 모든 몬스터들의 경험치 평균을 출력
dictionary.Count하면 key의 개수를 구한다
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class HelloWorld : MonoBehaviour
{
// Start is called before the first frame update
void Start()
{
Dictionary<string, int> exp = new Dictionary<string, int>();
exp.Add("슬라임", 2);
exp.Add("츄츄족", 4);
exp.Add("고래", 6);
int total = 0;
foreach (string key in exp.Keys)
{
total += exp[key];
}
Debug.Log(total / exp.Count);
}
// Update is called once per frame
void Update()
{
}
}
8. 연습문제7
서로 다른 뜻을 가진 한국어 : 영어 의 dictionary를 만들고 5개의 데이터를 넣는다.
이 dictionary를 영어: 한국어의 dictionary로 바꾼다
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class HelloWorld : MonoBehaviour
{
// Start is called before the first frame update
void Start()
{
Dictionary<string,string> KorToEng = new Dictionary<string,string>();
Dictionary<string,string> EngToKor = new Dictionary<string,string>();
KorToEng.Add("가", "a");
KorToEng.Add("나", "b");
KorToEng.Add("다", "c");
KorToEng.Add("라", "d");
KorToEng.Add("마", "e");
foreach (KeyValuePair<string,string> pair in KorToEng)
{
EngToKor[pair.Value] = pair.Key;
}
foreach (KeyValuePair<string, string> pair in EngToKor)
{
Debug.Log(pair.Key + pair.Value);
}
}
// Update is called once per frame
void Update()
{
}
}
9. 연습문제8
2*2 정수형 행렬 2개를 만들고 두 행렬의 합과 곱을 구한다.
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class HelloWorld : MonoBehaviour
{
// Start is called before the first frame update
void Start()
{
int[,] a =
{
{1,2 },
{3, 4 }
};
int[,] b =
{
{3, 4 },
{4,5 }
};
int[,] c = new int[2, 2];
int[,] d = new int[2, 2];
for(int i = 0; i < 2; i++)
{
for(int j = 0; j < 2; j++)
{
c[i, j] = a[i, j] + b[i, j];
}
}
for (int i = 0; i < 2; i++)
{
for(int j = 0; j < 2; j++)
{
int v = 0;
for(int k = 0; k < 2; k++)
{
v += a[i, k] * b[k, j];
}
d[i, j] = v;
}
}
}
// Update is called once per frame
void Update()
{
}
}
10. 연습문제9
배열에 아무렇게나 수를 넣고 오름차순 정렬해서 출력하기
https://deepdata.tistory.com/459
거품이 올라오는 것처럼 정렬하는 bubble sort(버블 정렬)
1. 개요 인접한 두개의 원소를 비교하면서 자리를 계속 교환하면서 정렬하는 알고리즘 첫번째 원소부터 인접한 원소끼리 계속 자리를 교환하면서 맨 마지막 자리까지 이동 한 단계가 끝나면, 가
deepdata.tistory.com
버블정렬을 직접 구현해보기
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class HelloWorld : MonoBehaviour
{
// Start is called before the first frame update
void Start()
{
int[] a = new int[5] { 3, 5, 2, 1, 4 };
for (int i = a.Length-1; i >= 0; i--)
{
for (int j = 0; j < i; j++)
{
if (a[j] > a[j + 1])
{
int b = a[j];
a[j] = a[j + 1];
a[j + 1] = b;
}
}
}
foreach(int n in a)
{
Debug.Log(n);
}
}
// Update is called once per frame
void Update()
{
}
}
'프로그래밍 > Unity&C#' 카테고리의 다른 글
Unity 기본9 - enum, struct (0) | 2024.04.23 |
---|---|
Unity 기본8 - C# 사용자 정의 함수 사용법 (0) | 2024.04.22 |
Unity 기본6 - C#의 컬렉션 List, Dictionary (0) | 2024.04.20 |
Unity 기본5 - C# 배열에 대해 (0) | 2024.04.19 |
Unity 기본4 - 반복문 while, for문 (0) | 2024.04.18 |